개발 과정 - 티스토리
개발 결과 - 깃허브
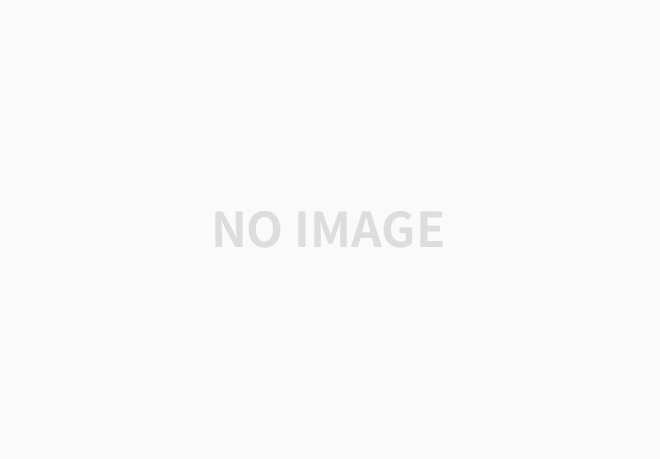
1. Insta 360 SDK
1.2 GPU 기반 하드웨어 가속
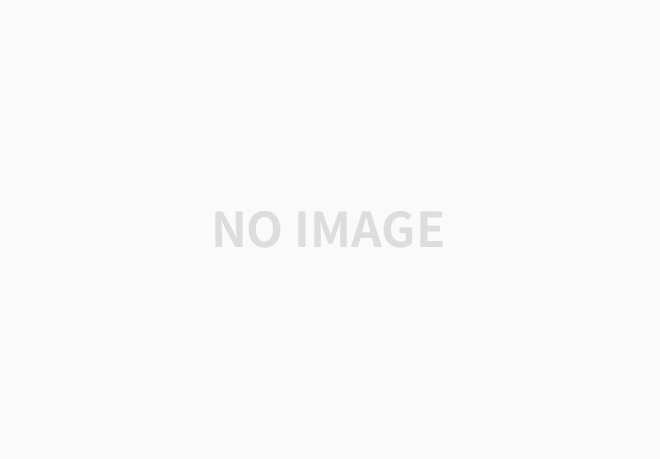
Insta360 카메라로부터 비디오 데이터를 스트리밍하고, FFmpeg을 활용해 하드웨어 가속을 통한 H.264 디코딩을 수행하며, NVIDIA의 CUDA 기반 디코딩을 지원한다. 또한, 디코딩된 데이터를 BMP 이미지로 저장하거나 원본 H.264 데이터를 파일로 저장하는 기능을 포함하고 있다.
주요 라이브러리 및 헤더
#include "Unity/IUnityGraphics.h"
#include <iostream>
#include "camera/camera.h"
#include "camera/device_discovery.h"
extern "C"
{
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/avutil.h>
#include <libavutil/hwcontext.h>
}
Unity/IUnityGraphics.h: Unity에서 사용할 수 있도록 플러그인을 작성할 때 필요한 헤더 파일이다. Unity는 C++로 작성된 플러그인을 호출할 수 있는데, 이를 위해 Unity의 렌더링과 관련된 API를 포함해야 한다.
camera/camera.h, camera/device_discovery.h: Insta360 카메라의 SDK를 사용하여 카메라 장치를 검색하고, 연결하고, 데이터를 수신하는 기능을 제공한다.
FFmpeg 관련 헤더 (libavcodec, libavformat, libavutil): 영상 및 오디오 데이터를 인코딩 및 디코딩하는 데 필요한 라이브러리다. 이 코드는 FFmpeg을 이용해 H.264 스트림을 하드웨어 가속을 통해 디코딩하는 기능을 수행한다.
Unity 내에서는 카메라의 원본 데이터를 직접 처리하기 어려우므로, 이 플러그인이 중간에서 데이터를 처리하고 Unity가 이해할 수 있는 형태로 변환하여 전달하는 역할을 한다.
전역 변수
static std::mutex g_ffmpegDecodeMutex;
static std::mutex g_codecContextMutex;
static std::array<AVCodecContext*, 2> g_AVCodecContexts = { nullptr, nullptr };
static std::shared_ptr<ins_camera::Camera> g_Camera = nullptr;
static std::shared_ptr<ins_camera::StreamDelegate> g_Delegate = nullptr;
static AVBufferRef* hw_device_ctx = NULL;
static enum AVPixelFormat hw_pix_fmt;
여러 개의 스레드가 접근할 수도 있기 때문에 std::mutex를 사용하여 동기화하고 있다.
g_ffmpegDecodeMutex: FFmpeg 디코딩 과정에서 동기화를 위해 사용된다. 영상 프레임을 여러 개 동시에 처리할 수 있도록 하는 과정에서, 하나의 프레임이 아직 처리되지 않았는데 새로운 프레임이 들어오는 경우를 방지한다.
g_codecContextMutex: AVCodecContext를 여러 개의 스레드에서 안전하게 사용할 수 있도록 보호하는 역할을 한다.
g_AVCodecContexts: 카메라에서 **두 개의 비디오 스트림(예: 좌/우 카메라)**을 제공하는 경우, 각각의 스트림을 디코딩할 FFmpeg의 디코더 컨텍스트를 저장하는 배열이다.
g_Camera: 카메라 장치를 제어하기 위한 객체.
g_Delegate: 카메라에서 들어오는 데이터를 받을 수 있도록 하는 객체.
hw_device_ctx: FFmpeg에서 하드웨어 가속을 사용하기 위한 GPU 컨텍스트.
hw_pix_fmt: GPU에서 사용할 픽셀 포맷.
Insta360 카메라에서 영상 데이터를 받아오면 두 개의 영상 스트림(좌/우)을 디코딩해야 하는데, 이때 각각을 담당하는 AVCodecContext가 필요하다. 또한, FFmpeg이 CUDA를 통해 GPU에서 영상을 디코딩하도록 설정하려면, hw_device_ctx를 통해 GPU 컨텍스트를 지정해야 한다.
1) 하드웨어 가속 및 FFmpeg 디코더 초기화
하드웨어 가속 디코더 초기화
static int ffmpeg_hw_decoder_init(AVCodecContext* ctx, const enum AVHWDeviceType type)
{
int err = 0;
if ((err = av_hwdevice_ctx_create(&hw_device_ctx, type, NULL, NULL, 0)) < 0) {
std::cerr << "[insta360][error] Failed to create HW device context.\n";
return err;
}
ctx->hw_device_ctx = av_buffer_ref(hw_device_ctx);
return err;
}
이 함수는 FFmpeg의 하드웨어 가속을 위한 컨텍스트를 초기화하는 역할을 한다.
- av_hwdevice_ctx_create()를 사용하여 CUDA를 이용한 GPU 디코딩 환경을 생성한다.
- 생성된 컨텍스트를 ctx->hw_device_ctx에 설정하여 이후 디코딩 과정에서 GPU를 사용하도록 설정한다.
CUDA를 이용해 GPU에서 H.264 영상을 빠르게 디코딩하려면, FFmpeg에 하드웨어 디코딩을 사용할 수 있도록 설정해야 한다. 만약 이 과정이 실패하면, CPU에서만 디코딩해야 하므로 성능이 크게 저하될 수 있다.
하드웨어 가속 픽셀 포맷 설정
static enum AVPixelFormat get_hw_format(AVCodecContext* ctx, const enum AVPixelFormat* pix_fmts)
{
const enum AVPixelFormat* p;
for (p = pix_fmts; *p != -1; p++) {
if (*p == hw_pix_fmt)
return *p;
}
std::cerr << "[insta360][error] Failed to get HW surface format.\n";
return AV_PIX_FMT_NONE;
}
이 함수는 FFmpeg에서 사용할 하드웨어 가속 픽셀 포맷을 결정하는 역할을 한다.
- FFmpeg은 다양한 하드웨어 가속을 지원하지만, 이 코드에서는 CUDA(NVDEC)를 사용한 hw_pix_fmt 포맷만 선택하도록 설정되어 있다.
- 만약 하드웨어 가속이 지원되지 않는 경우, 오류를 출력하고 디코딩을 진행하지 않는다.
NVIDIA GPU에서 h264_cuvid를 사용하여 디코딩할 경우, 지원되는 픽셀 포맷이 NV12일 수 있다. 이때 get_hw_format()을 통해 NV12 픽셀 포맷을 선택하여 GPU에서 디코딩하도록 설정할 수 있다.
int ffmpeg_decoder_init() {
enum AVHWDeviceType device_type = av_hwdevice_find_type_by_name("cuda");
if (device_type == AV_HWDEVICE_TYPE_NONE) {
std::cerr << "[insta360][error] CUDA device type not supported." << std::endl;
return -1;
}
for (int i = 0; i < 2; i++) {
const AVCodec* decoder = avcodec_find_decoder_by_name("h264_cuvid");
if (!decoder) return -1;
AVCodecContext* ctx = avcodec_alloc_context3(decoder);
ctx->get_format = get_hw_format;
if (ffmpeg_hw_decoder_init(ctx, device_type) < 0) return -1;
if (avcodec_open2(ctx, decoder, NULL) < 0) return -1;
std::lock_guard<std::mutex> lock(g_codecContextMutex);
g_AVCodecContexts[i] = ctx;
}
return 0;
}
이 함수는 두 개의 비디오 스트림(좌/우 카메라)에 대해 CUDA 기반의 FFmpeg 디코더를 초기화하는 역할을 한다.
2) FFmpeg을 이용한 비디오 데이터 디코딩
비디오 스트림을 하드웨어 가속을 이용해 디코딩하는 과정은 FFmpeg의 디코더를 활용하여 이루어진다. 이 과정에서는 영상 데이터가 GPU에서 직접 디코딩된 후, 적절한 픽셀 포맷(NV12)으로 변환된 다음 CPU에서 활용할 수 있는 형태로 변환되는 과정이 포함된다.
FFmpeg 패킷을 디코딩하는 함수
int ffmpeg_decode_packet(const uint8_t* data, size_t size, int stream_index)
{
std::lock_guard<std::mutex> lock(g_codecContextMutex);
if (stream_index < 0 || stream_index >= 2 || !g_AVCodecContexts[stream_index]) return -1;
AVCodecContext* codecCtx = g_AVCodecContexts[stream_index];
AVPacket* packet = av_packet_alloc();
packet->data = const_cast<uint8_t*>(data);
packet->size = static_cast<int>(size);
int ret = avcodec_send_packet(codecCtx, packet);
av_packet_free(&packet);
if (ret < 0) return ret;
while (ret >= 0) {
AVFrame* frame = av_frame_alloc();
ret = avcodec_receive_frame(codecCtx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
av_frame_free(&frame);
break;
}
if (frame->format == hw_pix_fmt) {
int stepBytes;
Npp8u* bgrData = nppiMalloc_8u_C3(frame->width, frame->height, &stepBytes);
NppiSize oSizeROI = { frame->width, frame->height };
NppStatus stat = nppiNV12ToRGB_709HDTV_8u_P2C3R(frame->data, frame->linesize[0], bgrData, frame->width * 3, oSizeROI);
QueueBGRData(bgrData, frame->width, frame->height, frame->width * 3, stream_index);
}
av_frame_free(&frame);
}
return ret;
}
이 함수는 H.264로 인코딩된 영상 데이터를 받아, GPU를 이용해 디코딩한 후, BGR 포맷으로 변환하는 역할을 한다.
실행 과정
- 뮤텍스를 이용한 스레드 동기화
여러 개의 스레드가 동시에 디코딩 요청을 보내는 경우를 대비해 std::lock_guard<std::mutex>를 사용하여 동기화한다. - FFmpeg 패킷 생성 및 전송
- av_packet_alloc()을 통해 새로운 FFmpeg 패킷을 생성.
- 입력 데이터를 packet->data에 할당하고, avcodec_send_packet()을 통해 디코딩 컨텍스트에 전송.
- 디코딩된 프레임을 받아서 처리
- avcodec_receive_frame()을 통해 디코딩된 프레임을 하나씩 받아옴.
- 만약 프레임이 GPU 기반의 픽셀 포맷(hw_pix_fmt)으로 저장되어 있다면, NVIDIA의 NPP(NVIDIA Performance Primitives) 라이브러리를 이용해 NV12 → RGB 변환 수행.
- 변환된 데이터를 QueueBGRData()를 호출하여 저장.
실제 예시
예를 들어, Unity에서 Insta360 카메라의 실시간 스트림을 받아 화면에 출력하려면, 디코딩된 데이터를 RGB 형태로 변환한 후 Unity가 사용할 수 있는 텍스처 형태로 전달해야 한다. 이 과정에서 ffmpeg_decode_packet()이 H.264 스트림을 해석하여 프레임 단위로 RGB 변환을 수행하는 역할을 한다.
3) BGR 데이터를 BMP 파일로 저장
void SaveBGRDataToBMP(const Npp8u* bgrData, int width, int height, int stride, const char* filename, int idx)
{
const int headerSize = 54;
int rowSize = ((width * 3 + 3) & ~3);
int fileSize = headerSize + rowSize * height;
unsigned char* bmpData = (unsigned char*)malloc(fileSize);
if (!bmpData) return;
memset(bmpData, 0, headerSize);
bmpData[0] = 'B';
bmpData[1] = 'M';
*(int*)&bmpData[2] = fileSize;
*(int*)&bmpData[10] = headerSize;
*(int*)&bmpData[14] = 40;
*(int*)&bmpData[18] = width;
*(int*)&bmpData[22] = -height;
*(short*)&bmpData[26] = 1;
*(short*)&bmpData[28] = 24;
*(int*)&bmpData[34] = 0;
unsigned char* hostBGR = (unsigned char*)malloc(height * stride);
if (!hostBGR) {
free(bmpData);
return;
}
cudaMemcpy(hostBGR, bgrData, height * stride, cudaMemcpyDeviceToHost);
for (int y = 0; y < height; y++) {
const unsigned char* srcRow = hostBGR + y * stride;
unsigned char* dstRow = bmpData + headerSize + y * rowSize;
for (int x = 0; x < width; x++) {
dstRow[x * 3 + 0] = srcRow[x * 3 + 0];
dstRow[x * 3 + 1] = srcRow[x * 3 + 1];
dstRow[x * 3 + 2] = srcRow[x * 3 + 2];
}
}
std::string finalPath = std::string(filename) + "_" + std::to_string(idx) + ".bmp";
FILE* file = fopen(finalPath.c_str(), "wb");
if (file) {
fwrite(bmpData, 1, fileSize, file);
fclose(file);
}
free(hostBGR);
free(bmpData);
}
이 함수는 디코딩된 BGR 데이터를 BMP 파일로 변환하여 저장하는 역할을 한다.
실행 과정
- BMP 헤더 생성
- BMP 파일은 54바이트 헤더 + 픽셀 데이터로 구성되며, 헤더에는 이미지 크기, 비트 깊이(24비트), 압축 여부 등의 정보가 포함된다.
- CUDA에서 BGR 데이터를 복사
- cudaMemcpy()를 사용하여 GPU에서 CPU 메모리로 데이터를 복사한다.
- 파일로 저장
- BMP 데이터를 생성하고 fwrite()를 사용해 파일로 저장.
실제 예시
예를 들어, Unity 내에서 특정 프레임을 스냅샷으로 저장하고 싶을 때, 이 함수가 사용될 수 있다. 사용자가 Unity에서 "현재 프레임 저장" 버튼을 누르면, 해당 프레임의 BGR 데이터를 BMP 파일로 변환하여 저장할 수 있다.
4) Unity에서 호출할 수 있는 함수
extern "C" void UNITY_INTERFACE_EXPORT UNITY_INTERFACE_API StartStream()
- 카메라를 찾아 연결하고 스트리밍을 시작한다.
- FFmpeg 디코더를 초기화하여 영상을 실시간으로 디코딩할 준비를 한다.
extern "C" void UNITY_INTERFACE_EXPORT UNITY_INTERFACE_API StopStream()
스트리밍을 중지하고, 디코더 및 하드웨어 컨텍스트를 해제한다.
extern "C" void UNITY_INTERFACE_EXPORT UNITY_INTERFACE_API StartSaveVideo()
원본 H.264 데이터를 파일로 저장할 수 있도록 활성화한다.
extern "C" void UNITY_INTERFACE_EXPORT UNITY_INTERFACE_API StopSaveVideo()
H.264 데이터 저장을 중지한다.
2. 어안 렌즈 360도 스티칭
3. 실시간 영상 텍스쳐 네트워크 동기화
3.1 머테리얼을 할당한 네트워크 오브젝트의 동기화
GPU 가속을 활용하여 Insta360에서 유니티로 받아지는 실시간 텍스처를 원격 사용자에게 제공하려면, 네트워크를 통해 이를 동기화하는 과정이 필요했다. 처음 시도한 방법은, 실시간 텍스처가 할당된 머티리얼을 Fusion2를 이용해 네트워크 오브젝트로 설정한 Sphere에 적용한 뒤, 이를 스폰하는 방식이었다. 그러나 이러한 방식으로는 실시간 텍스처 동기화가 제대로 이루어지지 않아 원하는 결과를 얻을 수 없었다. 원인을 분석해본 결과는 다음과 같았다.
1. 머티리얼은 CPU에서 관리되지만, 내부의 텍스처(Texture2D)는 GPU 메모리(GPU VRAM)에 로드된다.
Fusion2가 동기화할 수 있는 데이터는 일반적으로 CPU 메모리에 존재하는 직렬화 가능한 데이터인데, GPU VRAM에 위치한 텍스처 데이터는 네트워크 동기화가 불가능하다. 즉, 네트워크를 통해 객체(Sphere)와 머티리얼(Material)의 ID나 프로퍼티 값(예: 색상, float 값)은 동기화할 수 있어도, 텍스처(Texture2D)와 같은 대용량 GPU 리소스는 동기화되지 않는다.
2. 머티리얼 자체의 동기화 문제도 있다.
머티리얼은 Material 클래스의 인스턴스로 관리되며, 이 인스턴스는 개별 오브젝트마다 다를 수 있다.예를 들어, 원격 클라이언트가 오브젝트(Sphere)를 받아올 때 머티리얼을 복제(Clone)할 수도 있으며, 머티리얼 자체는 ID만 공유될 뿐, 내부의 텍스처는 공유되지 않는다.
따라서 실시간 텍스쳐의 네트워크 동기화를 가능하게 하기 위해서는, 텍스처를 CPU를 통해 압축해서 스트리밍 전송을 할 필요가 있었다. 그래서 생각한 방식은, GPU에서 렌더링된 텍스처 데이터를 CPU로 복사한 후 압축(Encoding)하고, 이를 네트워크를 통해 클라이언트로 전송한 뒤 복구(Decoding)하여 적용하는 방식이다. Fusion에서는 Remote Procedure Call (RPC)을 사용하여 서버에서 클라이언트로 데이터를 실시간으로 전달할 수 있기 때문에, 텍스쳐 정보를 바이트 배열로 변환한 후 RPC함수로 전송하는 것이다.
3.2 텍스처를 압축 후 RPC 함수를 통한 스트리밍 전송
1) 초기 로직
Fusion 네트워크 시스템을 활용하여 Texture2D 데이터를 압축 후 조각(Chunk) 단위로 전송하고, 클라이언트에서 이를 복구하여 실시간으로 동기화하는 기능을 구현했다. 서버는 주기적으로 텍스처의 변경 여부를 확인하고, 변경된 경우에만 압축하여 RPC를 통해 클라이언트에 전송한다. 클라이언트는 수신한 데이터를 복구하여 Texture2D로 변환한 뒤 머티리얼에 적용하는 방식이다.
주기적으로 텍스쳐 전송 트리거
void Update()
{
if (HasStateAuthority && Time.time - _lastSendTime > _sendInterval)
{
_lastSendTime = Time.time;
SendTextureData();
}
}
HasStateAuthority(서버 권한을 가진 경우)를 확인한 후, _sendInterval(0.1초)마다 SendTextureData()를 호출하여 텍스처를 전송하도록 한다. 이를 통해 서버는 불필요한 전송을 방지하면서 일정 주기마다 최신 텍스처 데이터를 감지하고 전송할 수 있다.
텍스처를 압축하여 네트워크 전송
void SendTextureData()
{
Texture2D updatedTexture = GetUpdatedTexture(sourceTexture);
if (_lastSentTexture != null && AreTexturesEqual(_lastSentTexture, updatedTexture))
{
Debug.Log("[Send] Texture not changed, skipping transmission.");
return;
}
_lastSentTexture = updatedTexture;
byte[] compressedTextureData = CompressTexture(updatedTexture);
_expectedSize = compressedTextureData.Length;
_receivedData.Clear();
Debug.Log($"[Send] Compressed Texture Size: {_expectedSize} bytes");
for (int i = 0; i < _expectedSize; i += ChunkSize)
{
int size = Math.Min(ChunkSize, _expectedSize - i);
byte[] chunk = new byte[size];
Array.Copy(compressedTextureData, i, chunk, 0, size);
RpcSendTextureChunk(chunk, _expectedSize);
}
}
이 함수는 먼저 GetUpdatedTexture(sourceTexture)를 호출하여 현재 텍스처 데이터를 복사한다. 이후 _lastSentTexture와 비교하여 동일한 경우에는 전송을 스킵하고, 변경이 감지된 경우에만 CompressTexture(updatedTexture)를 호출하여 PNG로 변환 후 GZip 압축을 수행한다. 압축된 데이터를 ChunkSize(480바이트) 단위로 나누어 RpcSendTextureChunk(chunk, _expectedSize)을 호출하여 네트워크로 전송한다. 이를 통해 대역폭을 효율적으로 사용하면서 데이터 손실 없이 클라이언트에 전달할 수 있다.
클라이언트에 조각(Chunk) 데이터 전송
[Rpc(RpcSources.StateAuthority, RpcTargets.All)]
void RpcSendTextureChunk(byte[] chunk, int totalSize)
{
if (_receivedData.Count == 0) _expectedSize = totalSize;
_receivedData.AddRange(chunk);
Debug.Log($"[Receive] Received {chunk.Length} bytes, Total: {_receivedData.Count}/{_expectedSize}");
if (_receivedData.Count >= _expectedSize)
{
Debug.Log("[Receive] All chunks received, applying texture.");
ApplyTexture(_receivedData.ToArray());
_receivedData.Clear();
}
}
서버에서 호출하는 이 RPC 함수는 클라이언트에서 텍스처 조각 데이터를 수신하는 역할을 한다. 첫 번째 청크가 도착하면 _expectedSize를 설정하여 전체 데이터 크기를 저장하고, 수신된 조각을 _receivedData 리스트에 추가한다. 모든 조각이 도착하면 ApplyTexture(_receivedData.ToArray())를 호출하여 압축 해제 후 텍스처를 복원하고, _receivedData를 초기화하여 다음 전송을 준비한다.
현재 텍스처를 새로운 텍스처로 복사
Texture2D GetUpdatedTexture(Texture2D original)
{
Texture2D newTexture = new Texture2D(original.width, original.height, TextureFormat.RGBA32, false);
RenderTexture rt = RenderTexture.GetTemporary(original.width, original.height);
Graphics.Blit(original, rt);
RenderTexture previous = RenderTexture.active;
RenderTexture.active = rt;
newTexture.ReadPixels(new Rect(0, 0, rt.width, rt.height), 0, 0);
newTexture.Apply();
RenderTexture.active = previous;
RenderTexture.ReleaseTemporary(rt);
return newTexture;
}
이 함수는 기존 Texture2D를 새로운 Texture2D로 복사하는 역할을 한다. RenderTexture를 이용하여 GPU에서 픽셀 데이터를 읽어 새로운 텍스처로 변환하며, 이는 텍스처를 GPU에서 CPU로 안전하게 가져오는 방식이다. 이를 통해 네트워크로 전송할 수 있는 데이터 형태로 변환할 수 있다.
두 텍스처가 동일한지 비교
bool AreTexturesEqual(Texture2D tex1, Texture2D tex2)
{
return StructuralComparisons.StructuralEqualityComparer.Equals(tex1.GetRawTextureData(), tex2.GetRawTextureData());
}
이 함수는 GetRawTextureData()를 이용하여 두 개의 텍스처 데이터를 비교하고 동일한 경우 true를 반환한다. 이를 통해 같은 데이터를 중복 전송하는 것을 방지하며, 네트워크 부하를 줄일 수 있다.
텍스처를 압축 후 바이트 배열 반환
byte[] CompressTexture(Texture2D texture)
{
byte[] textureBytes = texture.EncodeToPNG();
using (MemoryStream outputStream = new MemoryStream())
{
using (GZipStream compressionStream = new GZipStream(outputStream, CompressionMode.Compress))
{
compressionStream.Write(textureBytes, 0, textureBytes.Length);
}
return outputStream.ToArray();
}
}
이 함수는 EncodeToPNG()를 사용하여 Texture2D를 PNG 포맷의 바이트 배열로 변환한 뒤, GZipStream을 사용하여 데이터를 압축한다. 압축된 바이트 데이터를 반환하여 네트워크로 전송할 수 있도록 준비한다. 이 과정은 데이터 크기를 줄여 네트워크 대역폭을 절약하는 데 필수적이다.
수신한 텍스처 데이터를 복원
void ApplyTexture(byte[] compressedData)
{
byte[] decompressedData = DecompressData(compressedData);
Texture2D texture = new Texture2D(2, 2);
texture.LoadImage(decompressedData);
_objectRenderer.material.mainTexture = texture;
}
클라이언트가 수신한 압축된 데이터를 복원하는 함수이다. DecompressData()를 사용하여 압축을 해제한 후, Texture2D.LoadImage()를 이용해 Texture2D로 변환한다. 이후 _objectRenderer.material.mainTexture에 적용하여 실시간으로 텍스처를 업데이트한다.
압축 해제 후 바이트 배열 반환
private byte[] DecompressData(byte[] compressedData)
{
using (MemoryStream inputStream = new MemoryStream(compressedData))
using (GZipStream decompressionStream = new GZipStream(inputStream, CompressionMode.Decompress))
using (MemoryStream outputStream = new MemoryStream())
{
decompressionStream.CopyTo(outputStream);
return outputStream.ToArray();
}
}
이 함수는 GZipStream을 사용하여 압축된 바이트 배열을 원본 데이터로 복구하는 역할을 한다. 네트워크로 전송된 압축된 텍스처 데이터를 복원하여 클라이언트에서 사용할 수 있도록 한다.
하지만 이 로직도 제대로 동작하지 않았다. 원인을 조사해본 결과 다음과 같았다.
1. 네트워크 대역폭과 전송 속도의 한계
고해상도 텍스처(예: 1024x1024 이상)를 네트워크를 통해 주기적으로 전송하려면, 압축 후에도 상당한 양의 데이터를 주고받아야 한다. Texture2D.EncodeToPNG()를 사용하여 압축하면 파일 크기를 줄일 수 있지만, 고해상도일수록 PNG 데이터 자체도 크기가 커지며, 실시간 전송을 위한 충분한 네트워크 대역폭이 확보되지 않으면 패킷 지연, 데이터 손실 등의 문제가 발생할 수 있다.
2. EncodeToPNG() 또는 EncodeToJPG()의 성능 병목
Texture2D.EncodeToPNG()와 EncodeToJPG()는 CPU에서 실행되는 연산이므로, 높은 해상도의 텍스처를 자주 변환할 경우 CPU 사용량이 급격히 증가한다. PNG 또는 JPG로 인코딩하는 과정은 단순한 데이터 직렬화가 아니라 무손실/손실 압축 알고리즘을 적용하는 과정이 포함되므로, 해상도가 높아질수록 연산 부담이 커진다. 특히, Unity의 EncodeToPNG()는 멀티스레딩을 지원하지 않기 때문에 단일 프레임 내에서 실행될 경우 렌더링 속도를 저하시킬 가능성이 크다. 이는 게임의 성능 저하로 이어지고, 네트워크 전송 주기보다 더 오래 걸리는 경우, 최신 텍스처를 적시에 보내지 못해 실시간 동기화가 깨질 수 있다.
3. Fusion의 RPC Payload 크기 제한
Fusion 네트워크 시스템에서는 RPC를 통한 데이터 패킷 크기에 제한이 있다. 일반적으로, Fusion의 네트워크 메시지 크기는 몇 KB 수준으로 제한되므로, 큰 크기의 텍스처 데이터를 한 번에 전송할 수 없다. 이 코드에서는 ChunkSize = 480으로 설정하여 데이터를 나누어 전송하지만, 고해상도 텍스처의 경우 조각의 개수가 지나치게 많아져 패킷 오버헤드가 증가한다. 예를 들어, 1024x1024 해상도의 PNG 파일이 500KB 이상이라면, ChunkSize = 480을 기준으로 1000개 이상의 청크를 전송해야 한다. 이 과정에서 데이터 순서가 꼬이거나, 일부 패킷이 손실되었을 경우 텍스처 복원이 실패할 가능성이 있다.
전체 코드
using Fusion;
using System.IO.Compression;
using System.IO;
using System;
using System.Collections.Generic;
using UnityEngine;
using System.Collections;
public class TextureSync : NetworkBehaviour
{
public Renderer _objectRenderer; // 텍스처가 적용될 오브젝트
public Texture2D sourceTexture; // 외부 DLL이 변경하는 텍스처
float _sendInterval = 0.1f; // 0.5초마다 업데이트
float _sendInterval = 0.1f; // t초마다 업데이트
float _lastSendTime;
private const int ChunkSize = 480; // RPC Payload 제한 방지를 위해 512보다 작게 설정
private List<byte> _receivedData = new List<byte>(); // 수신 데이터 저장
private int _expectedSize = 0; // 예상되는 전체 데이터 크기
private Texture2D _lastSentTexture; // 마지막으로 전송한 텍스처 (중복 전송 방지)
void Update()
{
if (HasStateAuthority && Time.time - _lastSendTime > _sendInterval)
{
_lastSendTime = Time.time;
SendTextureData();
}
}
void SendTextureData()
{
// 최신 픽셀 데이터를 포함하는 Texture2D를 생성하여 비교
Texture2D updatedTexture = GetUpdatedTexture(sourceTexture);
// 아래 조건문은 필요 시 주석 및 해제
// 이전에 보낸 데이터와 현재 데이터를 비교하여 변경된 경우에만 전송
if (_lastSentTexture != null && AreTexturesEqual(_lastSentTexture, updatedTexture))
{
Debug.Log("[Send] Texture not changed, skipping transmission.");
return; // 텍스처가 변경되지 않았으면 전송하지 않음
}
_lastSentTexture = updatedTexture; // 현재 텍스처를 저장하여 다음 비교에 사용
byte[] compressedTextureData = CompressTexture(updatedTexture);
_expectedSize = compressedTextureData.Length;
_receivedData.Clear();
Debug.Log($"[Send] Compressed Texture Size: {_expectedSize} bytes");
for (int i = 0; i < _expectedSize; i += ChunkSize)
{
int size = Math.Min(ChunkSize, _expectedSize - i);
byte[] chunk = new byte[size];
Array.Copy(compressedTextureData, i, chunk, 0, size);
RpcSendTextureChunk(chunk, _expectedSize);
}
}
[Rpc(RpcSources.StateAuthority, RpcTargets.All)]
void RpcSendTextureChunk(byte[] chunk, int totalSize)
{
if (_receivedData.Count == 0) _expectedSize = totalSize; // 첫 번째 청크에서 전체 크기 설정
_receivedData.AddRange(chunk);
Debug.Log($"[Receive] Received {chunk.Length} bytes, Total: {_receivedData.Count}/{_expectedSize}");
if (_receivedData.Count >= _expectedSize)
{
Debug.Log("[Receive] All chunks received, applying texture.");
ApplyTexture(_receivedData.ToArray());
_receivedData.Clear(); // 적용 후 리스트 초기화
}
}
Texture2D GetUpdatedTexture(Texture2D original)
{
// 원본 텍스처를 새로운 Texture2D로 복사하여 픽셀 데이터를 가져옴
Texture2D newTexture = new Texture2D(original.width, original.height, TextureFormat.RGBA32, false);
RenderTexture rt = RenderTexture.GetTemporary(original.width, original.height);
Graphics.Blit(original, rt);
RenderTexture previous = RenderTexture.active;
RenderTexture.active = rt;
newTexture.ReadPixels(new Rect(0, 0, rt.width, rt.height), 0, 0);
newTexture.Apply();
RenderTexture.active = previous;
RenderTexture.ReleaseTemporary(rt);
return newTexture;
}
bool AreTexturesEqual(Texture2D tex1, Texture2D tex2)
{
return StructuralComparisons.StructuralEqualityComparer.Equals(tex1.GetRawTextureData(), tex2.GetRawTextureData());
}
byte[] CompressTexture(Texture2D texture)
{
byte[] textureBytes = texture.EncodeToPNG();
using (MemoryStream outputStream = new MemoryStream())
{
using (GZipStream compressionStream = new GZipStream(outputStream, CompressionMode.Compress))
{
compressionStream.Write(textureBytes, 0, textureBytes.Length);
}
return outputStream.ToArray();
}
}
void ApplyTexture(byte[] compressedData)
{
byte[] decompressedData = DecompressData(compressedData);
Texture2D texture = new Texture2D(2, 2);
texture.LoadImage(decompressedData);
_objectRenderer.material.mainTexture = texture;
}
private byte[] DecompressData(byte[] compressedData)
{
using (MemoryStream inputStream = new MemoryStream(compressedData))
using (GZipStream decompressionStream = new GZipStream(inputStream, CompressionMode.Decompress))
using (MemoryStream outputStream = new MemoryStream())
{
decompressionStream.CopyTo(outputStream);
return outputStream.ToArray();
}
}
}
2) 최적화
기존 코드 대비 개선된 점
1. AsyncGPUReadback을 활용하여 GPU → CPU 데이터 복사 최적화
private void RequestUpdatedTexture(Texture2D original, Action<Texture2D> callback, int scaleFactor = 2)
{
int targetWidth = original.width / scaleFactor;
int targetHeight = original.height / scaleFactor;
if (_renderTexture == null || _renderTexture.width != targetWidth || _renderTexture.height != targetHeight)
{
if (_renderTexture != null) _renderTexture.Release();
_renderTexture = new RenderTexture(targetWidth, targetHeight, 0, RenderTextureFormat.ARGB32);
_renderTexture.Create();
}
if (_updatedTexture == null || _updatedTexture.width != targetWidth || _updatedTexture.height != targetHeight)
{
_updatedTexture = new Texture2D(targetWidth, targetHeight, TextureFormat.RGBA32, false);
}
Graphics.Blit(original, _renderTexture);
AsyncGPUReadback.Request(_renderTexture, 0, request =>
{
if (request.hasError)
{
Debug.LogError("AsyncGPUReadback error");
callback(null);
return;
}
_updatedTexture.LoadRawTextureData(request.GetData<byte>());
_updatedTexture.Apply();
callback(_updatedTexture);
});
}
기존에는 Graphics.Blit()과 ReadPixels()을 이용해 GPU에서 CPU로 데이터를 복사했지만, AsyncGPUReadback을 사용하여 비동기 방식으로 데이터를 가져오도록 개선했다. 이를 통해 메인 스레드에서 블로킹이 발생하는 문제를 줄이고 실시간 성능을 향상시켰다.
운영 체제 관점에서 기존 방식의 문제점과 개선
기존 방식에서는 Graphics.Blit()을 사용해 GPU에서 RenderTexture로 화면을 복사한 후, ReadPixels()을 통해 CPU가 해당 데이터를 가져오는 방식이었다. 그러나 ReadPixels() 함수는 GPU가 처리한 데이터를 CPU 메모리(RAM)로 이동시키는 과정에서 강제로 대기(blocking)하도록 만든다. 운영 체제 관점에서 보면, GPU는 별도의 하드웨어 장치로 비동기적으로 연산을 수행하는 병렬 처리 장치다. 그런데 ReadPixels()을 호출하면 CPU는 GPU가 데이터를 제공할 때까지 기다려야 하며, 이 과정에서 게임의 메인 스레드(main thread)가 멈추는 문제가 발생한다.
운영 체제에서는 프로세스(게임 실행)와 스레드(게임 로직, 렌더링 등)를 일정한 시간 간격으로 실행하는 스케줄링 기법을 사용한다. 하지만 ReadPixels()이 실행되면 GPU가 데이터를 다 복사해줄 때까지 기다려야 하기 때문에 CPU는 다른 작업을 처리하지 못하고 멈추게 된다. 특히, 고해상도 텍스처를 가져올 때는 데이터 양이 많아져 이 대기 시간이 길어지며, 결국 프레임 속도(FPS)가 크게 떨어지는 문제가 발생한다.
이를 해결하기 위해 AsyncGPUReadback을 사용한다. 운영 체제의 비동기(Asynchronous) 실행 원리를 활용하여, CPU가 GPU 데이터를 요청한 후, 기다리지 않고 다른 작업을 수행할 수 있도록 변경하는 방식이다. 이 함수는 GPU에서 데이터를 읽어오는 과정에서 작업을 예약(Schedule)해두고, 데이터가 준비되면 자동으로 가져올 수 있도록 한다. 운영 체제 관점에서 보면, AsyncGPUReadback은 비동기 요청을 보내고, GPU가 작업을 완료하면 콜 (callback) 방식으로 결과를 CPU에게 전달하는 구조다. 즉, GPU가 데이터를 다 준비할 때까지 CPU는 멈추지 않고 다른 작업을 수행할 수 있으며, 데이터가 준비되면 자동으로 콜백을 통해 이를 받아와 적용하는 방식이다.
이 과정은 운영 체제의 I/O 비동기 처리 방식과 유사한 원리로 작동한다. 예를 들어, 파일을 읽거나 네트워크 요청을 보낼 때 CPU가 데이터를 기다리며 멈추는 것이 아니라, 요청만 보내고, 데이터가 준비되면 OS가 자동으로 CPU에 전달하는 방식과 동일하다.
추가적인 메모리 최적화 (RenderTexture 및 Texture2D 재사용)
운영 체제의 메모리 관리 원리 중 하나는 동적 할당(Dynamic Allocation)과 해제(Deallocation)이다. 기존 방식에서는 ReadPixels()을 사용할 때마다 새로운 Texture2D를 생성하고, 사용이 끝나면 이를 해제하는 방식이었다. 하지만, 이 방식은 불필요한 메모리 할당 및 해제를 반복하면서 Garbage Collector(GC)가 자주 동작하게 만들고, 이로 인해 프레임 드롭이 발생할 가능성이 커진다. 이를 해결하기 위해, RenderTexture와 Texture2D를 미리 생성해두고 재사용하는 방식으로 변경했다. 매번 새로운 메모리를 할당하는 것이 아니라, 일정 크기의 메모리를 미리 확보하고 이를 계속 사용하는 방식(Memory Pooling)과 유사하다. 이 방식은 불필요한 메모리 할당과 해제를 최소화하여, GC가 불필요하게 실행되지 않도록 하며, 메모리 사용량을 일정하게 유지할 수 있다. 결과적으로, 고해상도 텍스처에서도 불필요한 메모리 연산을 줄이고, 프레임 드롭 없이 안정적으로 데이터 업데이트가 가능해진다.
2. 텍스처 데이터를 타일(Tile) 단위로 전송하여 네트워크 부하 감소
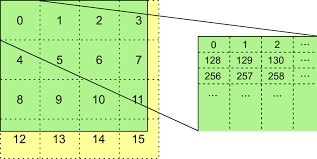
void SendTextureDataTiled(int textureIndex, Texture2D texture)
{
int textureWidth = texture.width;
int textureHeight = texture.height;
int tilesX = Mathf.CeilToInt(textureWidth / (float)TileSize);
int tilesY = Mathf.CeilToInt(textureHeight / (float)TileSize);
RpcSendTextureMeta(textureIndex, textureWidth, textureHeight, tilesX, tilesY, TileSize);
for (int ty = 0; ty < tilesY; ty++)
{
for (int tx = 0; tx < tilesX; tx++)
{
int startX = tx * TileSize;
int startY = ty * TileSize;
int currentTileWidth = Mathf.Min(TileSize, textureWidth - startX);
int currentTileHeight = Mathf.Min(TileSize, textureHeight - startY);
Color[] tilePixels = texture.GetPixels(startX, startY, currentTileWidth, currentTileHeight);
Texture2D tileTexture = new Texture2D(currentTileWidth, currentTileHeight, texture.format, false);
tileTexture.SetPixels(tilePixels);
tileTexture.Apply();
byte[] compressedTileData = CompressData(tileTexture);
int totalSize = compressedTileData.Length;
for (int i = 0; i < totalSize; i += ChunkSize)
{
int size = Math.Min(ChunkSize, totalSize - i);
byte[] chunk = new byte[size];
Array.Copy(compressedTileData, i, chunk, 0, size);
RpcSendTextureTileChunk(textureIndex, tx, ty, chunk, totalSize);
}
}
}
}
전체 텍스처를 한 번에 전송하는 것이 아니라, 512x512 크기의 타일(Tile) 단위로 분할하여 전송함으로써 네트워크 부하를 줄였다. 작은 단위로 쪼개서 보내기 때문에 Fusion의 RPC Payload 제한 문제를 해결하고, 손실된 타일만 다시 요청하는 방식으로 데이터 전송의 안정성을 높였다.
3. GZip 압축을 JPG로 변경하여 전송 데이터 크기 최적화
byte[] CompressTexture(Texture2D texture)
{
byte[] textureBytes = texture.EncodeToPNG();
using (MemoryStream outputStream = new MemoryStream())
{
using (GZipStream compressionStream = new GZipStream(outputStream, CompressionMode.Compress))
{
compressionStream.Write(textureBytes, 0, textureBytes.Length);
}
return outputStream.ToArray();
}
}
기존 방식에서는 PNG 인코딩 후 GZip으로 압축했지만, 이번에는 JPG(80% 품질)로 변환한 후 GZip 압축을 적용했다. JPG 자체가 손실 압축이므로, GZip을 추가로 적용했을 때 압축률이 더 높아져 네트워크 전송량이 줄어든다.
4, 전체 텍스처를 완전히 수신한 후 자동으로 조합(Reconstruct)
void ReconstructFullTexture(int textureIndex, TextureMeta meta)
{
Debug.Log($"[Reconstruct] Reconstructing full texture for Texture {textureIndex}");
Texture2D fullTexture = new Texture2D(meta.textureWidth, meta.textureHeight, TextureFormat.RGBA32, false);
Dictionary<(int, int), Texture2D> receivedTiles = (textureIndex == 1) ? receivedTiles1 : receivedTiles2;
foreach (var kvp in receivedTiles)
{
var (tileX, tileY) = kvp.Key;
Texture2D tile = kvp.Value;
int startX = tileX * meta.tileSize;
int startY = tileY * meta.tileSize;
fullTexture.SetPixels(startX, startY, tile.width, tile.height, tile.GetPixels());
}
fullTexture.Apply();
if (textureIndex == 1)
{
receivedTexture1 = fullTexture;
receivedTiles1.Clear();
}
else if (textureIndex == 2)
{
receivedTexture2 = fullTexture;
receivedTiles2.Clear();
}
}
각 타일을 개별적으로 전송하고, 모든 타일이 도착하면 ReconstructFullTexture()를 호출하여 원본 텍스처를 복원하는 방식으로 개선했다. 기존에는 한 번에 데이터를 받아야 했지만, 이제는 네트워크 환경이 불안정해도 개별 타일만 다시 요청하면 전체 데이터를 복원할 수 있다.
전체 코드
using Fusion;
using System.IO.Compression;
using System.IO;
using System;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Rendering;
using System.Collections;
public class TextureSync : NetworkBehaviour
{
public insta360 _instamanager;
[SerializeField] public Texture2D receivedTexture1;
[SerializeField] public Texture2D receivedTexture2;
// 전송 주기 (실시간이 아니어도 동작)
float _sendInterval = 0.5f;
float _lastSendTime;
// 청크 크기 (예: 300바이트)
private const int ChunkSize = 300;
// 타일 크기 (예: 512x512)
private const int TileSize = 512;
private Texture2D _lastSentTexture1;
private Texture2D _lastSentTexture2;
// 각 타일의 데이터를 임시 저장할 자료구조
class TileData
{
public int expectedSize;
public List<byte> receivedData = new List<byte>();
}
// 텍스처의 타일 전송 메타 정보와 타일별 데이터 저장소
class TextureMeta
{
public int textureWidth;
public int textureHeight;
public int tilesX;
public int tilesY;
public int tileSize;
public Dictionary<(int, int), TileData> tiles = new Dictionary<(int, int), TileData>();
}
private TextureMeta receivedMeta1;
private TextureMeta receivedMeta2;
// 타일 복원을 위한 수신된 타일 이미지 저장소
Dictionary<(int, int), Texture2D> receivedTiles1;
Dictionary<(int, int), Texture2D> receivedTiles2;
private RenderTexture _renderTexture;
private Texture2D _updatedTexture;
void Update()
{
if (HasStateAuthority && Time.time - _lastSendTime > _sendInterval)
}
}
// GPU로부터 텍스처 데이터를 비동기로 읽어옵니다.
private void RequestUpdatedTexture(Texture2D original, Action<Texture2D> callback, int scaleFactor = 2)
{
//AsyncGPUReadback.Request(original, 0, request =>
//{
// if (request.hasError)
// {
// Debug.LogError("AsyncGPUReadback error");
// callback(null);
// return;
// }
// Texture2D updatedTexture = new Texture2D(original.width, original.height, original.format, false);
// updatedTexture.LoadRawTextureData(request.GetData<byte>());
// updatedTexture.Apply();
// callback(updatedTexture);
//});
int targetWidth = original.width / scaleFactor;
int targetHeight = original.height / scaleFactor;
// GPU에서 다운스케일링
RenderTexture rt = RenderTexture.GetTemporary(targetWidth, targetHeight, 0, RenderTextureFormat.ARGB32);
Graphics.Blit(original, rt);
// 기존 RenderTexture를 재사용
if (_renderTexture == null || _renderTexture.width != targetWidth || _renderTexture.height != targetHeight)
{
if (_renderTexture != null) _renderTexture.Release();
// 비동기적 GPU → CPU 데이터 복사
AsyncGPUReadback.Request(rt, 0, request =>
_renderTexture = new RenderTexture(targetWidth, targetHeight, 0, RenderTextureFormat.ARGB32);
_renderTexture.Create();
}
// 기존 Texture2D 재사용
if (_updatedTexture == null || _updatedTexture.width != targetWidth || _updatedTexture.height != targetHeight)
{
_updatedTexture = new Texture2D(targetWidth, targetHeight, TextureFormat.RGBA32, false);
}
// GPU에서 다운스케일
Graphics.Blit(original, _renderTexture);
// 비동기적 GPU > CPU
AsyncGPUReadback.Request(_renderTexture, 0, request =>
{
if (request.hasError)
{
Debug.LogError("AsyncGPUReadback error");
RenderTexture.ReleaseTemporary(rt);
callback(null);
return;
}
// 원본 포맷 유지
Texture2D updatedTexture = new Texture2D(targetWidth, targetHeight, original.format, false);
updatedTexture.LoadRawTextureData(request.GetData<byte>());
updatedTexture.Apply();
_updatedTexture.LoadRawTextureData(request.GetData<byte>()); // Setpixels32 사용?
_updatedTexture.Apply();
RenderTexture.ReleaseTemporary(rt);
callback(updatedTexture);
callback(_updatedTexture);
});
}
// 데이터를 GZip으로 압축하는 함수 (PNG 등 인코딩된 데이터를 대상으로)
byte[] CompressData(Texture2D texture)
{
// JPG로 변환
byte[] textureBytes = texture.EncodeToJPG(80);
using (MemoryStream outputStream = new MemoryStream())
{
using (GZipStream compressionStream = new GZipStream(outputStream, CompressionMode.Compress))
{
compressionStream.Write(textureBytes, 0, textureBytes.Length);
}
return outputStream.ToArray();
}
}
// 텍스처 전체를 한 번에 전송하지 않고 타일 단위로 분할하여 전송합니다.
void SendTextureDataTiled(int textureIndex, Texture2D texture)
{
int textureWidth = texture.width;
int textureHeight = texture.height;
int tilesX = Mathf.CeilToInt(textureWidth / (float)TileSize);
int tilesY = Mathf.CeilToInt(textureHeight / (float)TileSize);
// 전송할 타일 메타 정보를 먼저 전송 (전체 텍스처 크기, 타일 개수 등)
RpcSendTextureMeta(textureIndex, textureWidth, textureHeight, tilesX, tilesY, TileSize);
// 각 타일별로 인코딩, 압축 후 청크 단위 전송
for (int ty = 0; ty < tilesY; ty++)
{
for (int tx = 0; tx < tilesX; tx++)
{
int startX = tx * TileSize;
int startY = ty * TileSize;
int currentTileWidth = Mathf.Min(TileSize, textureWidth - startX);
int currentTileHeight = Mathf.Min(TileSize, textureHeight - startY);
Color[] tilePixels = texture.GetPixels(startX, startY, currentTileWidth, currentTileHeight);
Texture2D tileTexture = new Texture2D(currentTileWidth, currentTileHeight, texture.format, false);
tileTexture.SetPixels(tilePixels);
tileTexture.Apply();
// PNG 인코딩 및 압축
//byte[] tilePNG = tileTexture.EncodeToPNG();
byte[] compressedTileData = CompressData(tileTexture);
int totalSize = compressedTileData.Length;
Debug.Log($"[Send{textureIndex}] Tile ({tx}, {ty}) Compressed Size: {totalSize} bytes");
// 압축된 데이터를 ChunkSize 단위로 분할하여 전송
for (int i = 0; i < totalSize; i += ChunkSize)
{
int size = Math.Min(ChunkSize, totalSize - i);
byte[] chunk = new byte[size];
Array.Copy(compressedTileData, i, chunk, 0, size);
RpcSendTextureTileChunk(textureIndex, tx, ty, chunk, totalSize);
}
}
}
}
// 수신측에서 타일 메타 정보를 수신합니다.
[Rpc(RpcSources.StateAuthority, RpcTargets.All)]
void RpcSendTextureMeta(int textureIndex, int textureWidth, int textureHeight, int tilesX, int tilesY, int tileSize)
{
Debug.Log($"[Meta] Received Meta for Texture {textureIndex}: {textureWidth}x{textureHeight}, Tiles: {tilesX}x{tilesY}, TileSize: {tileSize}");
TextureMeta meta = new TextureMeta()
{
textureWidth = textureWidth,
textureHeight = textureHeight,
tilesX = tilesX,
tilesY = tilesY,
tileSize = tileSize
};
if (textureIndex == 1)
{
receivedMeta1 = meta;
receivedTiles1 = new Dictionary<(int, int), Texture2D>();
}
else if (textureIndex == 2)
{
receivedMeta2 = meta;
receivedTiles2 = new Dictionary<(int, int), Texture2D>();
}
}
// 각 타일의 청크 데이터를 수신합니다.
[Rpc(RpcSources.StateAuthority, RpcTargets.All)]
void RpcSendTextureTileChunk(int textureIndex, int tileX, int tileY, byte[] chunk, int totalSize)
{
TextureMeta meta = (textureIndex == 1) ? receivedMeta1 : receivedMeta2;
if (meta == null)
{
Debug.LogError($"[Receive{textureIndex}] Texture meta not received yet.");
return;
}
// 해당 타일의 데이터 저장소를 가져오거나 새로 생성합니다.
var key = (tileX, tileY);
if (!meta.tiles.ContainsKey(key))
{
meta.tiles[key] = new TileData() { expectedSize = totalSize };
}
TileData tileData = meta.tiles[key];
tileData.receivedData.AddRange(chunk);
Debug.Log($"[Receive{textureIndex}] Tile ({tileX}, {tileY}) Received {chunk.Length} bytes, Total: {tileData.receivedData.Count}/{tileData.expectedSize}");
if (tileData.receivedData.Count >= tileData.expectedSize)
{
Debug.Log($"[Receive{textureIndex}] Tile ({tileX}, {tileY}) fully received, processing tile.");
ProcessReceivedTile(textureIndex, tileX, tileY, tileData.receivedData.ToArray());
meta.tiles.Remove(key);
// 모든 타일을 수신했다면 전체 텍스처를 재구성
if (meta.tiles.Count == 0)
{
ReconstructFullTexture(textureIndex, meta);
}
}
}
// 개별 타일 데이터를 복원하여 Texture2D로 생성
void ProcessReceivedTile(int textureIndex, int tileX, int tileY, byte[] compressedData)
{
byte[] decompressedData = DecompressData(compressedData);
Texture2D tileTexture = new Texture2D(2, 2);
tileTexture.LoadImage(decompressedData);
if (textureIndex == 1)
{
if (receivedTiles1 == null) receivedTiles1 = new Dictionary<(int, int), Texture2D>();
receivedTiles1[(tileX, tileY)] = tileTexture;
}
else if (textureIndex == 2)
{
if (receivedTiles2 == null) receivedTiles2 = new Dictionary<(int, int), Texture2D>();
receivedTiles2[(tileX, tileY)] = tileTexture;
}
}
// 압축 해제
private byte[] DecompressData(byte[] compressedData)
{
using (MemoryStream inputStream = new MemoryStream(compressedData))
using (GZipStream decompressionStream = new GZipStream(inputStream, CompressionMode.Decompress))
using (MemoryStream outputStream = new MemoryStream())
{
decompressionStream.CopyTo(outputStream);
return outputStream.ToArray();
}
}
// 모든 타일 데이터를 수신한 후, 전체 텍스처를 재구성합니다.
void ReconstructFullTexture(int textureIndex, TextureMeta meta)
{
Debug.Log($"[Reconstruct] Reconstructing full texture for Texture {textureIndex}");
Texture2D fullTexture = new Texture2D(meta.textureWidth, meta.textureHeight, TextureFormat.RGBA32, false);
Dictionary<(int, int), Texture2D> receivedTiles = (textureIndex == 1) ? receivedTiles1 : receivedTiles2;
if (receivedTiles == null)
{
Debug.LogError($"[Reconstruct] No received tiles for Texture {textureIndex}");
return;
}
// 각 타일의 픽셀 데이터를 전체 텍스처에 복사
foreach (var kvp in receivedTiles)
{
var (tileX, tileY) = kvp.Key;
Texture2D tile = kvp.Value;
int startX = tileX * meta.tileSize;
int startY = tileY * meta.tileSize;
Color[] tilePixels = tile.GetPixels();
fullTexture.SetPixels(startX, startY, tile.width, tile.height, tilePixels);
}
fullTexture.Apply();
if (textureIndex == 1)
{
receivedTexture1 = fullTexture;
Debug.Log("[Reconstruct] Texture 1 reconstructed.");
receivedTiles1.Clear();
}
else if (textureIndex == 2)
{
receivedTexture2 = fullTexture;
Debug.Log("[Reconstruct] Texture 2 reconstructed.");
receivedTiles2.Clear();
}
}
// 기존 텍스처 비교 (메모리의 원시 데이터 비교)
bool AreTexturesEqual(Texture2D tex1, Texture2D tex2)
{
return StructuralComparisons.StructuralEqualityComparer.Equals(tex1.GetRawTextureData(), tex2.GetRawTextureData());
}
}
이처럼 시도해볼만한 최적화는 모두 시도해보았지만 여전히 문제가 해결되지 않았다. 이에 대해 조사해보니, Fusion 자체가 Transform 동기화와 게임 상태 동기화에 최적화된 네트워크 엔진이지만, 낮은 주기로 대량의 데이터를 전송하는 데는 적합하지 않은 것같다. 특히, 비디오 프레임처럼 지속적으로 변하는 데이터를 매 프레임마다 송수신하는 경우 비효율적이며, 이로인해 실시간 스트리밍에 필요한 높은 전송 속도와 안정성을 보장하기 어려운 것이다. 결국 새로운 방안을 찾아야만 했다.
3.3 실시간 영상 스트리밍
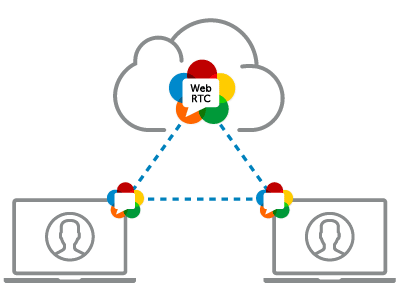
이러한 문제를 해결하기 위해 게임 엔진의 동기화는 Fusion2로, 실시간 영상 전송에는 WebRTC를 이용하여 Hybrid 방식을 도입하기로 결정했다. WebRTC는 비디오 프레임처럼 지속적으로 변하는 데이터를 효율적으로 송수신할 수 있어 실시간 스트리밍에 적합하다. Fusion은 게임 상태 동기화에는 최적화되어 있지만, 대량의 데이터를 매 프레임마다 전송하는 데 비효율적이었으며, 이로 인해 전송 속도와 안정성을 보장하기 어려웠다. 따라서 두 방식을 혼합하여 원격 협업 환경을 구축하고자 했다.
WebRTC는 P2P 기반으로 작동하기 때문에 네트워크 품질이 변동될 경우 대역폭 관리와 패킷 손실 보정이 제한적일 수 있으며, 특히 고해상도 영상의 지속적인 전송에서는 지연이나 프레임 드롭이 발생할 가능성이 크다. 조사 결과, Agora Video SDK를 이용하면 적응형 비트레이트 기술을 통해 네트워크 상태에 따라 자동으로 영상 품질을 조정하며, 자체 글로벌 중계 서버를 활용해 보다 안정적이고 끊김 없는 고해상도 영상 스트리밍을 이용할 수 있었다. 또한, 고성능 오디오 및 비디오 코덱 최적화가 적용되어 있어 동일한 네트워크 환경에서도 WebRTC보다 더 높은 해상도와 프레임 속도를 유지할 수 있다. 이러한 특성 덕분에 실시간 스트리밍의 품질이 중요한 환경에서는 Agora Video SDK가 보다 효과적인 솔루션이 될 수 있는 것이다. 공식 문서를 보고 다음과 같은 과정에 기반해 코드를 설계했다.
전체 실행 흐름
- 앱 시작 → App ID 검사 → Insta360 연결 대기
- Agora 엔진 초기화 → 이벤트 핸들러 등록
- 커스텀 비디오 트랙 생성
- 채널 입장 (Host 역할 설정)
- 매 프레임 Insta360 Texture → Agora 전송
- 원격 사용자 UI 생성·제거
- 앱 종료 시 Agora 리소스 정리
- Agora 엔진 초기화는 SDK 내부의 오디오·비디오 캡처, 인코딩, 네트워크 모듈 등을 메모리에 로드하고, App ID 기반 인증, 디바이스 권한 확보, 이벤트 루프 설정 등 핵심 기능을 사용할 수 있도록 준비하는 과정이다. 초기화 시점에 RTC 엔진 인스턴스가 생성되며, 내부적으로는 스레드 풀 구성, 네이티브 리소스 할당, 플랫폼별 시스템 콜 연결이 이루어지기 때문에, 이를 생략하면 이후 API 호출이 실패하거나 비정상 동작이 발생할 수 있다.
전체 코드
1️⃣ Start(): 초기 검증 & Insta360 연결 대기
if (CheckAppId())
{
StartCoroutine(InitializeAfterInsta360Connected());
InitEngine();
CreateCustomVideoTrack();
JoinChannel();
}
- CheckAppId(): App ID 길이 검사 → 유효하지 않으면 로그 출력
- InitializeAfterInsta360Connected(): _insta360.isConnected 가 true 될 때까지 대기 → RawImage에 Texture 할당
2️⃣ InitEngine(): Agora 엔진 준비
RtcEngine = RtcEngine.CreateAgoraRtcEngineEx();
RtcEngine.Initialize(new RtcEngineContext { appId = _appID, channelProfile = CHANNEL_PROFILE_TYPE.LIVE_BROADCASTING });
RtcEngine.InitEventHandler(new UserEventHandlerInsta(this));
- RtcEngineContext: 라이브 방송 모드, 오디오/비디오 시나리오 설정
- UserEventHandlerInsta: Join/Leave/UserJoined/UserOffline 콜백 처리
3️⃣ CreateCustomVideoTrack(): 트랙 생성
_videoTrack = RtcEngine.CreateCustomVideoTrack();
Log.UpdateLog($"CustomVideoTrack ID: {_videoTrack}");
Agora가 관리하는 “빈 비디오 스트림” 생성 → ID 반환
4️⃣ JoinChannel(): 채널 입장 (방송 시작)
RtcEngine.EnableAudio();
RtcEngine.EnableVideo();
RtcEngine.SetClientRole(CLIENT_ROLE_TYPE.CLIENT_ROLE_BROADCASTER);
var options = new ChannelMediaOptions {
publishCameraTrack = false,
publishCustomVideoTrack = true,
customVideoTrackId = _videoTrack
};
RtcEngine.JoinChannel(_token, _channelName, 0, options);
- CLIENT_ROLE_BROADCASTER: 송출자 권한
- 기본 카메라 트랙 비활성 → 커스텀 트랙 전송
5️⃣ ShareScreen(): 매 프레임 영상 Push
yield return new WaitForEndOfFrame();
var extFrame = new ExternalVideoFrame {
type = VIDEO_BUFFER_TYPE.VIDEO_BUFFER_TEXTURE,
textureId = _insta360.frontTexture.GetNativeTexturePtr().ToInt32(),
stride = width, height = height,
rotation = 180,
timestamp = DateTime.Now.Ticks/10000
};
RtcEngine.PushVideoFrame(extFrame, _videoTrack);
- ExternalVideoFrame: GPU 텍스처 → Agora (Zero‑copy 전송)
- 매 프레임 호출 (EndOfFrame 이후)
6️⃣ 원격 사용자 화면 관리
OnUserJoined → MakeVideoView(uid)
- UID 기반 GameObject 생성 → Canvas 자식
- RawImage + VideoSurface 추가 → 자동 크기 조정
OnUserOffline → DestroyVideoView(uid)
- 해당 UID GameObject 삭제
7️⃣ OnDestroy(): 리소스 정리
RtcEngine.DestroyCustomVideoTrack(_videoTrack);
RtcEngine.LeaveChannel();
RtcEngine.Dispose();
커스텀 트랙 삭제 → 채널 탈퇴 → 엔진 Dispose
하지만 여기에는 두 가지 문제를 간과했다. 아고라에서는 영상 전송에서 기본적으로 다음 두 가지 기능을 제공한다.
1. 실시간 영상의 Raw 데이터를 자체적으로 인코딩 후 네트워크로 전달
2. 저장된 h.264 영상 데이터를 스트리밍
즉, 이미 인코딩된 형태의 실시간 h.264를 전송할 때는 다음과 같은 문제가 발생했다.
1. WebRTC는 그냥 바이트 배열을 받지 않음
- WebRTC는 영상 파일처럼 던져주면 재생해주는 구조가 아님.
- 대신 RTP 포맷이라는 네트워크 전송용 포맷을 기대함.
- 즉, 영상 데이터 포장을 제대로 해야 WebRTC가 알아듣는다.
2. H.264는 포장과 정보가 복잡함
- H.264는 프레임 앞에 SPS, PPS, IDR 같은 메타정보가 있어야 재생 가능.
- 실시간이면 순서와 타이밍도 맞아야 하고, IDR 없으면 영상 깨짐.
- 그냥 H.264 바이트를 던지면, 상대는 "이게 뭔데?" 하고 못 읽는다.
3. 브라우저/WebRTC는 H.264 규칙도 타이트함
- 브라우저는 특정 프로파일(H.264 Baseline 등)만 지원.
- 인코딩된 영상이 이걸 안 맞추면, 재생 안 됨.
📽️ 1. 영상 데이터(Video Data)
영상 데이터는 시간의 흐름에 따라 연속적으로 표시되는 이미지들의 집합이다. 하나의 영상은 수많은 정지 이미지(프레임)로 구성되며, 이 이미지들이 빠르게 재생됨으로써 움직이는 장면처럼 보이게 된다. 이 데이터는 색상, 해상도, 프레임 속도 등 다양한 속성을 포함하며, 이를 통해 영상의 품질과 용량이 결정된다.
🎞️ 2. H.264
H.264는 고화질 영상 데이터를 효율적으로 압축하기 위한 비디오 압축 표준이다. 원본 영상의 품질은 최대한 유지하면서 데이터 용량을 크게 줄일 수 있어, 저장 공간을 절약하고 전송 속도를 빠르게 한다. 이 코덱은 유튜브, 스마트폰, 방송, CCTV 등에서 널리 사용되며, 현재 가장 보편적인 영상 압축 기술 중 하나이다. H.264는 프레임 간의 중복 정보를 제거하는 방식 등을 통해 효율적인 압축을 실현한다.
🧩 3. 텍스처(Texture)
텍스처는 3D 그래픽이나 게임에서 물체 표면의 디테일을 표현하기 위해 사용하는 2D 이미지이다. 회색 또는 단순한 형태의 3D 모델에 텍스처를 입히면, 현실감 있는 색상, 무늬, 질감을 표현할 수 있게 된다.
텍스처는 피부, 옷감, 금속, 나무 등 다양한 재질을 표현하는 데 사용되며, 게임이나 영화에서 시각적 리얼리티를 높이는 중요한 요소이다.
3.4 h.264 원본 바이트 데이터 네트워크 전송
1) UDP
2) TCP
🧠 GPU 메모리와 CPU 힙 메모리는 어떻게 다를까?
일반적으로 프로그램은 데이터를 CPU 메모리(RAM) 에 저장하여 처리한다. C++에서 malloc, new 등을 사용하여 메모리를 동적으로 할당하면 이 메모리는 힙(heap) 영역에 생성된다. 하지만 GPU는 별도의 그래픽 전용 메모리(VRAM) 를 사용하며, 영상 처리나 색 변환 같은 연산은 대부분 GPU 메모리에서 직접 수행된다. 그래서 CUDA나 NPP 라이브러리를 사용할 경우, GPU가 사용할 수 있는 메모리를 따로 할당해야 한다.
📏 Stride(스트라이드)와 Padding(패딩)은 왜 생기나?
stride는 한 줄의 픽셀 데이터를 GPU 메모리에 저장할 때 실제로 사용되는 바이트 수를 의미한다. 예를 들어, 1280픽셀 너비의 이미지를 RGB(3바이트)로 저장하려면 이론적으로는 1280 × 3 = 3,840바이트가 필요하다. 하지만 GPU는 연산 속도를 높이기 위해 행의 끝에 추가 공간(패딩) 을 붙이며, 이 정렬 덕분에 GPU는 데이터를 더 빠르게 읽고 쓸 수 있다. NPP 라이브러리의 nppiMalloc_8u_C3() 함수로 GPU 메모리를 할당하면, 이 함수는 실제 할당된 stride 값을 함께 반환한다. 예를 들어 stride가 3,856바이트로 반환되었다면, GPU는 3,840바이트의 픽셀 데이터 외에 16바이트의 여분 공간을 추가한 것이다.
🧨 stride를 무시하면 어떤 문제가 생기는가?
stride를 무시하고 단순히 width × 3 = 3,840바이트만큼만 복사하면 문제가 발생한다. 실제로 GPU 메모리는 각 줄마다 3,856바이트를 할당했는데, 복사 작업은 그보다 16바이트 적게 진행되므로 줄과 줄 사이의 패딩 공간을 건너뛰지 못하게 된다. 그 결과, 다음 줄 복사가 잘못된 위치에서 시작되며 패딩 공간을 침범하여 메모리 오염이 발생하게 된다. 이런 덮어쓰기가 매 줄마다 반복되면, 1280줄 이미지 기준으로 16바이트 × 1280줄 = 20,480바이트가 잘못된 위치에 기록된다. 즉, GPU에서 받은 데이터를 복사하면서 CPU 힙 메모리의 다른 데이터나 구조를 침범하게 되는 것이다.
💥 힙(Heap) 메모리는 무엇이며, 왜 손상이 위험한가?
힙은 C++에서 malloc이나 new로 할당되는 동적 메모리 영역이다. 이 영역은 프로그램 실행 중 필요한 만큼 할당하거나 해제할 수 있도록 설계되어 있으며, 내부적으로는 메모리 블록 정보, 크기, 상태 등을 관리하는 메타데이터가 존재한다. 하지만 복사 범위가 잘못되어 이 영역의 경계를 침범하게 되면, 이러한 메타데이터가 덮어쓰여 메모리 할당과 해제 과정에서 예기치 않은 오류가 발생하게 된다. 특히 Unity처럼 C++과 C# 메모리 모델이 혼합된 복잡한 구조에서는, 이런 힙 손상이 바로 크래시, 렌더링 실패, Null 참조 에러 등으로 이어진다.
✅ stride를 사용하는 올바른 방식은 무엇인가?
이 문제를 해결하는 핵심은 GPU가 실제로 메모리를 어떻게 할당했는지를 고려하여 코드를 작성하는 것이다. nppiMalloc_8u_C3() 함수는 GPU가 할당한 정확한 stride 값을 알려준다. 이 값을 기준으로 cudaMemcpy()나 NPP 색 변환 함수에 전달하여 메모리 경계를 정확히 지킬 수 있다. 예를 들어 stride가 3,856바이트이고 이미지 높이가 1280줄이라면, 복사해야 할 전체 크기는 3,856 × 1280 = 4,933,120바이트이다. 이 값을 기준으로 복사하면, 패딩까지 포함한 GPU 메모리를 정확히 덮기 때문에 메모리 손상이 발생하지 않는다. Unity로 전송할 데이터 역시 이 stride 값을 기준으로 큐에 등록하면 안전하게 렌더링할 수 있다.
🔚 결론
Stride는 GPU가 메모리를 효율적으로 접근하기 위해 설정한 실제 한 줄의 메모리 크기이다. 이를 무시하고 단순히 width × 3으로만 계산하면 GPU가 붙인 패딩을 침범하게 되어 버퍼 오버런이 발생한다. 이로 인해 CPU 힙 메모리까지 손상되며, Unity 같은 환경에서는 바로 크래시로 이어지게 된다. 따라서 nppiMalloc_8u_C3() 같은 함수를 통해 정확한 stride 값을 얻고, 이를 cudaMemcpy와 이미지 처리 함수에서 반드시 사용해야 한다. 이것이 영상 처리 시스템에서 메모리 안전성과 성능을 동시에 보장하는 올바른 방식이다.
int ffmpeg_decode_packet(const uint8_t* data, size_t size, int stream_index)
{
std::lock_guard<std::mutex> lock(g_codecContextMutex);
if (stream_index < 0 || stream_index >= 2 || !g_AVCodecContexts[stream_index]) {
std::cerr << "[insta360][error] Invalid stream index: " << stream_index << "\n";
return -1;
}
AVCodecContext* codecCtx = g_AVCodecContexts[stream_index];
//codecCtx->hw_device_ctx = av_buffer_ref(g_HwDeviceCtxs[stream_index]);
AVPacket* packet = av_packet_alloc();
if (!packet) return AVERROR(ENOMEM);
packet->data = const_cast<uint8_t*>(data);
packet->size = static_cast<int>(size);
int ret = avcodec_send_packet(codecCtx, packet);
av_packet_free(&packet);
if (ret < 0) {
std::cerr << "[insta360][error] Error sending packet for decoding.\n";
return ret;
}
// 디코딩된 프레임을 반복적으로 받아옴
while (ret >= 0) {
AVFrame* frame = av_frame_alloc();
if (!frame) {
std::cerr << "[insta360][error] Failed to allocate frame.\n";
ret = AVERROR(ENOMEM);
break;
}
ret = avcodec_receive_frame(codecCtx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
av_frame_free(&frame);
break;
}
else if (ret < 0) {
std::cerr << "[insta360][error] Error during decoding.\n";
av_frame_free(&frame);
break;
}
// 하드웨어 픽셀 포맷이면
if (frame->format == hw_pix_fmt) {
int stepBytes;
Npp8u* bgrData = nullptr;
// Allocate memory for BGR data on the device
int stride;
bgrData = nppiMalloc_8u_C3(frame->width, frame->height, &stride);
if (!bgrData)
{
std::cerr << "[insta360][error] nppiMalloc_8u_C3 failed to allocate memory." << "\n";
return -1;
}
NppiSize oSizeROI = { frame->width,frame->height };
//DBUG_PRINT("width: %d, height: %d, stepBytes: %d\n", oSizeROI.width, oSizeROI.height, stepBytes);
// NppStatus stat = nppiNV12ToBGR_8u_P2C3R(frame_dec->data, frame_dec->width, bgrData, frame_dec->width*3*sizeof(Npp8u), oSizeROI);
#ifdef USE_709frame->data
NppStatus stat = nppiNV12ToBGR_8u_P2C3R(frame->data, frame->linesize[0], bgrData, stride, roi);
#else
NppStatus stat = nppiNV12ToRGB_709HDTV_8u_P2C3R(frame->data, frame->linesize[0], bgrData, frame->width * 3, oSizeROI);
#endif
//SaveBGRDataToBMP(bgrData, frame->width, frame->height, frame->width * 3, g_Delegate->GetSavePath(), stream_index);
QueueBGRData(bgrData, frame->width, frame->height, frame->width * 3, stream_index);
}
else
{
std::cout << "[insta360][warning] hw format is not NV12 format. It is " << frame->format << "\n";
}
av_frame_free(&frame);
}
return ret;
}
bgrData = nppiMalloc_8u_C3(frame->width, frame->height, &stepBytes);
NppStatus stat = nppiNV12ToBGR_8u_P2C3R(frame->data, frame->linesize[0], bgrData, frame->width * 3, oSizeROI);
- 너비(width)가 1280 픽셀이니, 한 줄에 필요한 RGB 데이터는1280 × 3 = 3,840바이트
- 이 계산값을 그대로 stride(한 줄당 바이트 수)로 사용했다.
- 하지만 GPU(NPP)는 메모리 접근 속도를 높이기 위해 각 줄 끝에 padding(여분 공간)을 붙인다. 이 예시에서는 실제로 3,856바이트를 할당한다.
- 결과적으로, 한 줄에 3,840바이트만 복사하고 나머지 16바이트는 건너뛰어 버퍼의 다음 줄 첫 부분을 덮어쓴다.
- 이 덮어쓰기가 매 줄마다 반복되어 총 16바이트 × 1280줄 = 20,480바이트가 힙 메모리에 손상된다.
- 힙 손상이 누적되면 Unity가 예기치 않은 메모리 영역을 읽거나 쓸 때 크래시가 발생한다.
int stride;
bgrData = nppiMalloc_8u_C3(frame->width, frame->height, &stride);
NppStatus stat = nppiNV12ToBGR_8u_P2C3R(frame->data, frame->linesize[0], bgrData, stride, roi);